Tool Calling Agent
Author: Kenny Jung
Peer Review:
Proofread : Chaeyoon Kim
This is a part of LangChain Open Tutorial
Overview
This tutorial explains tool calling in LangChain, allowing models to detect when one or more tools are called and what inputs to pass to those tools.
When making API calls, you can define tools and intelligently guide the model to generate structured objects, such as JSON, containing arguments for calling these tools.
The goal of the tools API is to provide more reliable generation of valid and useful tool calls beyond what standard text completion or chat APIs can achieve.
You can create agents that iteratively call tools and receive results until a query is resolved by integrating this structured output with the ability to bind multiple tools to a tool-calling chat model and letting the model choose which tools to call.
This represents a more generalized version of the OpenAI tools agent which was specifically designed for OpenAI's particular tool-calling style.
This agent uses LangChain's ToolCall interface to support a broader spectrum of provider implementations beyond OpenAI, including Anthropic
, Google Gemini
, and Mistral
.
Table of Contents
References

Environment Setup
Set up the environment. You may refer to Environment Setup for more details.
[Note]
langchain-opentutorial
is a package that provides a set of easy-to-use environment setup, useful functions and utilities for tutorials.You can checkout the
langchain-opentutorial
for more details.
%%capture --no-stderr
%pip install langchain-opentutorial
# Install required packages
from langchain_opentutorial import package
package.install(
[
"langsmith",
"langchain",
"langchain_core",
"langchain_openai",
"langchain_community",
],
verbose=False,
upgrade=False,
)
# Set environment variables
from langchain_opentutorial import set_env
set_env(
{
"OPENAI_API_KEY": "",
"LANGCHAIN_API_KEY": "",
"LANGCHAIN_TRACING_V2": "true",
"LANGCHAIN_ENDPOINT": "https://api.smith.langchain.com",
"LANGCHAIN_PROJECT": "ToolCallingAgent",
}
)
Environment variables have been set successfully.
You can alternatively set OPENAI_API_KEY
in .env
file and load it.
[Note] This is not necessary if you've already set OPENAI_API_KEY
in previous steps.
from dotenv import load_dotenv
load_dotenv(override=True)
True
Creating Tools
LangChain allows you to define custom tools that your agents can interact with. You can create tools for searching news or executing Python code.
The @tool
decorator is used to create tools:
TavilySearchResults
is a tool for searching news.PythonREPL
is a tool for executing Python code.
from langchain.tools import tool
from typing import List, Dict, Annotated
from langchain_community.tools import TavilySearchResults
from langchain_experimental.utilities import PythonREPL
# Creating tool for searching news
@tool
def search_news(query: str) -> List[Dict[str, str]]:
"""Search news by input keyword using Tavily Search API"""
news_tool = TavilySearchResults(
max_results=3,
include_answer=True,
include_raw_content=True,
include_images=True,
# search_depth="advanced",
# include_domains = [],
# exclude_domains = []
)
return news_tool.invoke(query, k=3)
# Creating tool for executing python code
@tool
def python_repl_tool(
code: Annotated[str, "The python code to execute to generate your chart."],
):
"""Use this tool to execute Python code. If you want to see the output of a value,
you should print it using print(...). This output is visible to the user."""
result = ""
try:
result = PythonREPL().run(code)
except BaseException as e:
print(f"Failed to execute. Error: {repr(e)}")
finally:
return result
print(f"Tool name: {search_news.name}")
print(f"Tool description: {search_news.description}")
print(f"Tool name: {python_repl_tool.name}")
print(f"Tool description: {python_repl_tool.description}")
Tool name: search_news
Tool description: Search news by input keyword using Tavily Search API
Tool name: python_repl_tool
Tool description: Use this tool to execute Python code. If you want to see the output of a value,
you should print it using print(...). This output is visible to the user.
# Creating tools
tools = [search_news, python_repl_tool]
Constructing an Agent Prompt
chat_history
: This variable stores the conversation history if your agent supports multi-turn. (Otherwise, you can omit this.)agent_scratchpad
: This variable serves as temporary storage for intermediate variables.input
: This variable represents the user's input.
from langchain_core.prompts import ChatPromptTemplate
# Creating prompt
# Prompt is a text that describes the task the model should perform. (input the name and role of the tool)
prompt = ChatPromptTemplate.from_messages(
[
(
"system",
"You are a helpful assistant. "
"Make sure to use the `search_news` tool for searching keyword related news.",
),
("placeholder", "{chat_history}"),
("human", "{input}"),
("placeholder", "{agent_scratchpad}"),
]
)
Creating Agent
Define an agent using the create_tool_calling_agent
function.
from langchain_openai import ChatOpenAI
from langchain.agents import create_tool_calling_agent
# Creating LLM
llm = ChatOpenAI(model="gpt-4o-mini", temperature=0)
# Creating Agent
agent = create_tool_calling_agent(llm, tools, prompt)
AgentExecutor
AgentExecutor
The AgentExecutor
is a class for managing an agent that uses tools.
Key properties
agent
: the underlying agent responsible for creating plans and determining actions at each step of the execution loop.tools
: a list containing all the valid tools that the agent is authorized to use.return_intermediate_steps
: boolean flag determins whether to return the intermediate steps the agent took along with the final output.max_iterations
: a maximum number of steps the agent can take before the execution loop is terminated.max_execution_time
: the maximum amount of time the execution loop is allowed to run.early_stopping_method
: a defined method how to handle situations when the agent does not return anAgentFinish
. ("force" or "generate")"force"
: returns a string indicating that the execution loop was stopped due to reaching the time or iteration limit."generate"
: calls the agent's LLM chain once to generate a final answer based on the previous steps taken.
handle_parsing_errors
: a specification how to handle parsing errors. (You can setTrue
,False
, or provide a custom error handling function.)trim_intermediate_steps
: method of trimming intermediate steps. (You can set-1
to keep all steps, or provide a custom trimming function.)
Key methods
invoke
: Executes the agent.stream
: Stream the steps required to reach the final output.
Key features
Tool validation : Ensure that the tool is compatible with the agent.
Execution control : Set maximum interations and execution time limits to manage agent bahavior.
Error handling : Offers various processing options for output parsing errors.
Intermediate step management : Allows for trimming intermediate steps or returning options for debugging.
Asynchronous support : Supports asynchronous execution and streaming of results.
Optimization tips
Set appropriate values for
max_iterations
andmax_execution_time
to manage execution time.Use
trim_intermediate_steps
to optimize memory usage.For complex tasks, use the
stream
method to monitor step-by-step results.
from langchain.agents import AgentExecutor
# Create AgentExecutor
agent_executor = AgentExecutor(
agent=agent,
tools=tools,
verbose=True,
max_iterations=10,
max_execution_time=10,
handle_parsing_errors=True,
)
# Run AgentExecutor
result = agent_executor.invoke({"input": "Search news about AI Agent in 2025."})
print("Agent execution result:")
print(result["output"])
> Entering new AgentExecutor chain...
Invoking: `search_news` with `{'query': 'AI Agent 2025'}`
[{'url': 'https://www.analyticsvidhya.com/blog/2024/12/ai-agent-trends/', 'content': 'In a similar study, Deloitte forecasts that 25% of enterprises using GenAI will deploy AI Agents by 2025, growing to 50% by 2027. Meanwhile, Gartner predicts that by 2028, at least 15% of day-to-day work decisions will be made autonomously through agentic AI. It also states that by then, 33% of enterprise software applications will also include'}, {'url': 'https://www.techtarget.com/searchEnterpriseAI/feature/Next-year-will-be-the-year-of-AI-agents', 'content': 'Next year will be the year of AI agents | TechTarget This will make the AI agent more accurate in completing its task, Greene said. Other than the rise of single-task AI agents, 2025 may also be the year of building the infrastructure for AI agents, said Olivier Blanchard, an analyst with Futurum Group. "2025 isn\'t going to be the year when we see a fully developed agentic AI," he said. AI agents need an orchestration layer that works across different platforms and devices, Blanchard said. Because data is usually spread across different sources and processes, it might be challenging to give AI agents the data they need to perform the tasks they\'re being asked to do, Greene said.'}, {'url': 'https://hai.stanford.edu/news/predictions-ai-2025-collaborative-agents-ai-skepticism-and-new-risks', 'content': 'According to leading experts from Stanford Institute for Human-Centered AI, one major trend is the rise of collaborative AI systems where multiple specialized agents work together, with humans providing high-level guidance. I expect to see more focus on multimodal AI models in education, including in processing speech and images. AI Agents Work Together In 2025, we will see a significant shift from relying on individual AI models to using systems where multiple AI agents of diverse expertise work together. As an example, we recently introduced the\xa0Virtual Lab, where a professor AI agent leads a team of AI scientist agents (e.g., AI chemist, AI biologist) to tackle challenging, open-ended research, with a human researcher providing high-level feedback. We will experience an emerging paradigm of research around how humans work together with AI agents.'}]Here are some recent news articles discussing the future of AI agents in 2025:
1. **AI Agent Trends**
- **Source**: [Analytics Vidhya](https://www.analyticsvidhya.com/blog/2024/12/ai-agent-trends/)
- **Summary**: A study by Deloitte forecasts that 25% of enterprises using Generative AI will deploy AI agents by 2025, with this number expected to grow to 50% by 2027. Gartner predicts that by 2028, at least 15% of day-to-day work decisions will be made autonomously through agentic AI.
2. **Next Year Will Be the Year of AI Agents**
- **Source**: [TechTarget](https://www.techtarget.com/searchEnterpriseAI/feature/Next-year-will-be-the-year-of-AI-agents)
- **Summary**: Analysts suggest that 2025 may focus on building the infrastructure necessary for AI agents. While single-task AI agents will rise, the development of an orchestration layer that works across various platforms and devices will be crucial for their effectiveness.
3. **Predictions for AI in 2025**
- **Source**: [Stanford Institute for Human-Centered AI](https://hai.stanford.edu/news/predictions-ai-2025-collaborative-agents-ai-skepticism-and-new-risks)
- **Summary**: Experts predict a shift towards collaborative AI systems where multiple specialized agents work together, guided by humans. This includes the development of multimodal AI models in education and research, where AI agents collaborate on complex tasks with human oversight.
These articles highlight the anticipated growth and evolution of AI agents, emphasizing collaboration, infrastructure development, and the integration of AI into enterprise decision-making processes.
> Finished chain.
Agent execution result:
Here are some recent news articles discussing the future of AI agents in 2025:
1. **AI Agent Trends**
- **Source**: [Analytics Vidhya](https://www.analyticsvidhya.com/blog/2024/12/ai-agent-trends/)
- **Summary**: A study by Deloitte forecasts that 25% of enterprises using Generative AI will deploy AI agents by 2025, with this number expected to grow to 50% by 2027. Gartner predicts that by 2028, at least 15% of day-to-day work decisions will be made autonomously through agentic AI.
2. **Next Year Will Be the Year of AI Agents**
- **Source**: [TechTarget](https://www.techtarget.com/searchEnterpriseAI/feature/Next-year-will-be-the-year-of-AI-agents)
- **Summary**: Analysts suggest that 2025 may focus on building the infrastructure necessary for AI agents. While single-task AI agents will rise, the development of an orchestration layer that works across various platforms and devices will be crucial for their effectiveness.
3. **Predictions for AI in 2025**
- **Source**: [Stanford Institute for Human-Centered AI](https://hai.stanford.edu/news/predictions-ai-2025-collaborative-agents-ai-skepticism-and-new-risks)
- **Summary**: Experts predict a shift towards collaborative AI systems where multiple specialized agents work together, guided by humans. This includes the development of multimodal AI models in education and research, where AI agents collaborate on complex tasks with human oversight.
These articles highlight the anticipated growth and evolution of AI agents, emphasizing collaboration, infrastructure development, and the integration of AI into enterprise decision-making processes.
Checking step-by-step results using Stream output
We will use the stream()
method of AgentExecutor
to stream the intermediate steps of the agent.
The output of stream()
alternates between (Action, Observation) pairs, and finally ends with the agent's answer if the goal is achieved.
The flow will look like the followings:
Action output
Observation output
Action output
Observation output
... (Continue until the goal is achieved) ...
Then, the agent will conclude a final answer if its goal is achieved.
The following table summarizes the content you'll encounter in the output:
Action
actions
: Represents the AgentAction
or its subclass.
messages
: Chat messages corresponding to the action call.
Observation
steps
: A record of the agent's work, including the current action and its observation.
messages
: Chat messages containing the results from function calls (i.e., observations).
Final Answer
output
: Represents AgentFinish
signal.
messages
: Chat messages containing the final output.
```python
from langchain.agents import AgentExecutor
# Create AgentExecutor
agent_executor = AgentExecutor(
agent=agent,
tools=tools,
verbose=False,
handle_parsing_errors=True,
)
# Run in streaming mode
result = agent_executor.stream({"input": "Search news about AI Agent in 2025."})
for step in result:
# Print intermediate steps
print(step)
print("===" * 20)
{'actions': [ToolAgentAction(tool='search_news', tool_input={'query': 'AI Agent 2025'}, log="\nInvoking: `search_news` with `{'query': 'AI Agent 2025'}`\n\n\n", message_log=[AIMessageChunk(content='', additional_kwargs={'tool_calls': [{'index': 0, 'id': 'call_xxsGXV6JZgYb7JAb0JU9EQ7o', 'function': {'arguments': '{"query":"AI Agent 2025"}', 'name': 'search_news'}, 'type': 'function'}]}, response_metadata={'finish_reason': 'tool_calls', 'model_name': 'gpt-4o-mini-2024-07-18', 'system_fingerprint': 'fp_72ed7ab54c'}, id='run-fc21b730-a5ac-4f0a-b009-585acca6519c', tool_calls=[{'name': 'search_news', 'args': {'query': 'AI Agent 2025'}, 'id': 'call_xxsGXV6JZgYb7JAb0JU9EQ7o', 'type': 'tool_call'}], tool_call_chunks=[{'name': 'search_news', 'args': '{"query":"AI Agent 2025"}', 'id': 'call_xxsGXV6JZgYb7JAb0JU9EQ7o', 'index': 0, 'type': 'tool_call_chunk'}])], tool_call_id='call_xxsGXV6JZgYb7JAb0JU9EQ7o')], 'messages': [AIMessageChunk(content='', additional_kwargs={'tool_calls': [{'index': 0, 'id': 'call_xxsGXV6JZgYb7JAb0JU9EQ7o', 'function': {'arguments': '{"query":"AI Agent 2025"}', 'name': 'search_news'}, 'type': 'function'}]}, response_metadata={'finish_reason': 'tool_calls', 'model_name': 'gpt-4o-mini-2024-07-18', 'system_fingerprint': 'fp_72ed7ab54c'}, id='run-fc21b730-a5ac-4f0a-b009-585acca6519c', tool_calls=[{'name': 'search_news', 'args': {'query': 'AI Agent 2025'}, 'id': 'call_xxsGXV6JZgYb7JAb0JU9EQ7o', 'type': 'tool_call'}], tool_call_chunks=[{'name': 'search_news', 'args': '{"query":"AI Agent 2025"}', 'id': 'call_xxsGXV6JZgYb7JAb0JU9EQ7o', 'index': 0, 'type': 'tool_call_chunk'}])]}
============================================================
{'steps': [AgentStep(action=ToolAgentAction(tool='search_news', tool_input={'query': 'AI Agent 2025'}, log="\nInvoking: `search_news` with `{'query': 'AI Agent 2025'}`\n\n\n", message_log=[AIMessageChunk(content='', additional_kwargs={'tool_calls': [{'index': 0, 'id': 'call_xxsGXV6JZgYb7JAb0JU9EQ7o', 'function': {'arguments': '{"query":"AI Agent 2025"}', 'name': 'search_news'}, 'type': 'function'}]}, response_metadata={'finish_reason': 'tool_calls', 'model_name': 'gpt-4o-mini-2024-07-18', 'system_fingerprint': 'fp_72ed7ab54c'}, id='run-fc21b730-a5ac-4f0a-b009-585acca6519c', tool_calls=[{'name': 'search_news', 'args': {'query': 'AI Agent 2025'}, 'id': 'call_xxsGXV6JZgYb7JAb0JU9EQ7o', 'type': 'tool_call'}], tool_call_chunks=[{'name': 'search_news', 'args': '{"query":"AI Agent 2025"}', 'id': 'call_xxsGXV6JZgYb7JAb0JU9EQ7o', 'index': 0, 'type': 'tool_call_chunk'}])], tool_call_id='call_xxsGXV6JZgYb7JAb0JU9EQ7o'), observation=[{'url': 'https://www.analyticsvidhya.com/blog/2024/12/ai-agent-trends/', 'content': 'In a similar study, Deloitte forecasts that 25% of enterprises using GenAI will deploy AI Agents by 2025, growing to 50% by 2027. Meanwhile, Gartner predicts that by 2028, at least 15% of day-to-day work decisions will be made autonomously through agentic AI. It also states that by then, 33% of enterprise software applications will also include'}, {'url': 'https://www.techtarget.com/searchEnterpriseAI/feature/Next-year-will-be-the-year-of-AI-agents', 'content': 'Next year will be the year of AI agents | TechTarget This will make the AI agent more accurate in completing its task, Greene said. Other than the rise of single-task AI agents, 2025 may also be the year of building the infrastructure for AI agents, said Olivier Blanchard, an analyst with Futurum Group. "2025 isn\'t going to be the year when we see a fully developed agentic AI," he said. AI agents need an orchestration layer that works across different platforms and devices, Blanchard said. Because data is usually spread across different sources and processes, it might be challenging to give AI agents the data they need to perform the tasks they\'re being asked to do, Greene said.'}, {'url': 'https://hai.stanford.edu/news/predictions-ai-2025-collaborative-agents-ai-skepticism-and-new-risks', 'content': 'According to leading experts from Stanford Institute for Human-Centered AI, one major trend is the rise of collaborative AI systems where multiple specialized agents work together, with humans providing high-level guidance. I expect to see more focus on multimodal AI models in education, including in processing speech and images. AI Agents Work Together In 2025, we will see a significant shift from relying on individual AI models to using systems where multiple AI agents of diverse expertise work together. As an example, we recently introduced the\xa0Virtual Lab, where a professor AI agent leads a team of AI scientist agents (e.g., AI chemist, AI biologist) to tackle challenging, open-ended research, with a human researcher providing high-level feedback. We will experience an emerging paradigm of research around how humans work together with AI agents.'}])], 'messages': [FunctionMessage(content='[{"url": "https://www.analyticsvidhya.com/blog/2024/12/ai-agent-trends/", "content": "In a similar study, Deloitte forecasts that 25% of enterprises using GenAI will deploy AI Agents by 2025, growing to 50% by 2027. Meanwhile, Gartner predicts that by 2028, at least 15% of day-to-day work decisions will be made autonomously through agentic AI. It also states that by then, 33% of enterprise software applications will also include"}, {"url": "https://www.techtarget.com/searchEnterpriseAI/feature/Next-year-will-be-the-year-of-AI-agents", "content": "Next year will be the year of AI agents | TechTarget This will make the AI agent more accurate in completing its task, Greene said. Other than the rise of single-task AI agents, 2025 may also be the year of building the infrastructure for AI agents, said Olivier Blanchard, an analyst with Futurum Group. \\"2025 isn\'t going to be the year when we see a fully developed agentic AI,\\" he said. AI agents need an orchestration layer that works across different platforms and devices, Blanchard said. Because data is usually spread across different sources and processes, it might be challenging to give AI agents the data they need to perform the tasks they\'re being asked to do, Greene said."}, {"url": "https://hai.stanford.edu/news/predictions-ai-2025-collaborative-agents-ai-skepticism-and-new-risks", "content": "According to leading experts from Stanford Institute for Human-Centered AI, one major trend is the rise of collaborative AI systems where multiple specialized agents work together, with humans providing high-level guidance. I expect to see more focus on multimodal AI models in education, including in processing speech and images. AI Agents Work Together In 2025, we will see a significant shift from relying on individual AI models to using systems where multiple AI agents of diverse expertise work together. As an example, we recently introduced the\xa0Virtual Lab, where a professor AI agent leads a team of AI scientist agents (e.g., AI chemist, AI biologist) to tackle challenging, open-ended research, with a human researcher providing high-level feedback. We will experience an emerging paradigm of research around how humans work together with AI agents."}]', additional_kwargs={}, response_metadata={}, name='search_news')]}
============================================================
{'output': 'Here are some recent news articles discussing the future of AI agents in 2025:\n\n1. **AI Agent Trends** \n - **Source**: [Analytics Vidhya](https://www.analyticsvidhya.com/blog/2024/12/ai-agent-trends/) \n - **Summary**: A study by Deloitte forecasts that 25% of enterprises using Generative AI will deploy AI agents by 2025, with this number expected to grow to 50% by 2027. Gartner predicts that by 2028, at least 15% of daily work decisions will be made autonomously through agentic AI.\n\n2. **Next Year Will Be the Year of AI Agents** \n - **Source**: [TechTarget](https://www.techtarget.com/searchEnterpriseAI/feature/Next-year-will-be-the-year-of-AI-agents) \n - **Summary**: Analysts suggest that 2025 may focus on building the infrastructure necessary for AI agents. While single-task AI agents will rise, the development of a comprehensive orchestration layer across platforms will be crucial for their effectiveness.\n\n3. **Predictions for AI in 2025** \n - **Source**: [Stanford Institute for Human-Centered AI](https://hai.stanford.edu/news/predictions-ai-2025-collaborative-agents-ai-skepticism-and-new-risks) \n - **Summary**: Experts predict a shift towards collaborative AI systems where multiple specialized agents work together, guided by humans. This includes the development of multimodal AI models in education and research, where AI agents collaborate on complex tasks.\n\nThese articles highlight the anticipated growth and evolution of AI agents, emphasizing their increasing integration into enterprise operations and collaborative environments.', 'messages': [AIMessage(content='Here are some recent news articles discussing the future of AI agents in 2025:\n\n1. **AI Agent Trends** \n - **Source**: [Analytics Vidhya](https://www.analyticsvidhya.com/blog/2024/12/ai-agent-trends/) \n - **Summary**: A study by Deloitte forecasts that 25% of enterprises using Generative AI will deploy AI agents by 2025, with this number expected to grow to 50% by 2027. Gartner predicts that by 2028, at least 15% of daily work decisions will be made autonomously through agentic AI.\n\n2. **Next Year Will Be the Year of AI Agents** \n - **Source**: [TechTarget](https://www.techtarget.com/searchEnterpriseAI/feature/Next-year-will-be-the-year-of-AI-agents) \n - **Summary**: Analysts suggest that 2025 may focus on building the infrastructure necessary for AI agents. While single-task AI agents will rise, the development of a comprehensive orchestration layer across platforms will be crucial for their effectiveness.\n\n3. **Predictions for AI in 2025** \n - **Source**: [Stanford Institute for Human-Centered AI](https://hai.stanford.edu/news/predictions-ai-2025-collaborative-agents-ai-skepticism-and-new-risks) \n - **Summary**: Experts predict a shift towards collaborative AI systems where multiple specialized agents work together, guided by humans. This includes the development of multimodal AI models in education and research, where AI agents collaborate on complex tasks.\n\nThese articles highlight the anticipated growth and evolution of AI agents, emphasizing their increasing integration into enterprise operations and collaborative environments.', additional_kwargs={}, response_metadata={})]}
============================================================
Customizing intermediate step output using user-defined functions
You can define the following 3 functions to customize the intermediate steps output:
tool_callback
: This function handles the output generated by tool calls.observation_callback
: This function deals with the observation data output.result_callback
: This function allows you to handle the final answer output.
Here's an example callback function that demonstrates how to clean up the intermediate steps of the Agent.
This callback function can be useful when presenting intermediate steps to users in an application like Streamlit.
from typing import Dict, Any
# Create AgentStreamParser class
class AgentStreamParser:
def __init__(self):
pass
def tool_callback(self, tool: Dict[str, Any]) -> None:
print("\n=== Tool Called ===")
print(f"Tool: {tool.get('tool')}")
print(f"Input: {tool.get('tool_input')}")
print("==================\n")
def observation_callback(self, step: Dict[str, Any]) -> None:
print("\n=== Observation ===")
observation_data = step["steps"][0].observation
print(f"Observation: {observation_data}")
print("===================\n")
def result_callback(self, result: str) -> None:
print("\n=== Final Answer ===")
print(result)
print("====================\n")
def process_agent_steps(self, step: Dict[str, Any]) -> None:
if "actions" in step:
for action in step["actions"]:
self.tool_callback(
{"tool": action.tool, "tool_input": action.tool_input}
)
elif "output" in step:
self.result_callback(step["output"])
else:
self.observation_callback(step)
# Create AgentStreamParser instance
agent_stream_parser = AgentStreamParser()
Check the response process of your Agent in streaming mode.
# Run in streaming mode
result = agent_executor.stream({"input": "Generate a pie chart using matplotlib."})
# result = agent_executor.stream({"input": "Search news about AI Agent in 2025."})
for step in result:
agent_stream_parser.process_agent_steps(step)
Python REPL can execute arbitrary code. Use with caution.

=== Tool Called ===
Tool: python_repl_tool
Input: {'code': "import matplotlib.pyplot as plt\n\n# Data to plot\nlabels = ['A', 'B', 'C', 'D']\nsizes = [15, 30, 45, 10]\ncolors = ['gold', 'yellowgreen', 'lightcoral', 'lightskyblue']\nexplode = (0.1, 0, 0, 0) # explode 1st slice\n\n# Plot\nplt.figure(figsize=(8, 6))\nplt.pie(sizes, explode=explode, labels=labels, colors=colors,\n autopct='%1.1f%%', shadow=True, startangle=140)\nplt.axis('equal') # Equal aspect ratio ensures that pie is drawn as a circle.\nplt.title('Pie Chart Example')\nplt.show()"}
==================
=== Observation ===
Observation:
===================
=== Final Answer ===
The pie chart has been generated successfully. However, I cannot display the chart directly here. You can run the provided code in your local Python environment to see the pie chart. Here’s the code again for your convenience:
```python
import matplotlib.pyplot as plt
# Data to plot
labels = ['A', 'B', 'C', 'D']
sizes = [15, 30, 45, 10]
colors = ['gold', 'yellowgreen', 'lightcoral', 'lightskyblue']
explode = (0.1, 0, 0, 0) # explode 1st slice
# Plot
plt.figure(figsize=(8, 6))
plt.pie(sizes, explode=explode, labels=labels, colors=colors,
autopct='%1.1f%%', shadow=True, startangle=140)
plt.axis('equal') # Equal aspect ratio ensures that pie is drawn as a circle.
plt.title('Pie Chart Example')
plt.show()
```
Feel free to modify the data and labels as needed!
====================
# Run in streaming mode
result = agent_executor.stream({"input": "Search news about AI Agent in 2025."})
for step in result:
agent_stream_parser.process_agent_steps(step)
=== Tool Called ===
Tool: search_news
Input: {'query': 'AI Agent 2025'}
==================
=== Observation ===
Observation: [{'url': 'https://www.analyticsvidhya.com/blog/2024/12/ai-agent-trends/', 'content': 'In a similar study, Deloitte forecasts that 25% of enterprises using GenAI will deploy AI Agents by 2025, growing to 50% by 2027. Meanwhile, Gartner predicts that by 2028, at least 15% of day-to-day work decisions will be made autonomously through agentic AI. It also states that by then, 33% of enterprise software applications will also include'}, {'url': 'https://www.techtarget.com/searchEnterpriseAI/feature/Next-year-will-be-the-year-of-AI-agents', 'content': 'Next year will be the year of AI agents | TechTarget This will make the AI agent more accurate in completing its task, Greene said. Other than the rise of single-task AI agents, 2025 may also be the year of building the infrastructure for AI agents, said Olivier Blanchard, an analyst with Futurum Group. "2025 isn\'t going to be the year when we see a fully developed agentic AI," he said. AI agents need an orchestration layer that works across different platforms and devices, Blanchard said. Because data is usually spread across different sources and processes, it might be challenging to give AI agents the data they need to perform the tasks they\'re being asked to do, Greene said.'}, {'url': 'https://hai.stanford.edu/news/predictions-ai-2025-collaborative-agents-ai-skepticism-and-new-risks', 'content': 'According to leading experts from Stanford Institute for Human-Centered AI, one major trend is the rise of collaborative AI systems where multiple specialized agents work together, with humans providing high-level guidance. I expect to see more focus on multimodal AI models in education, including in processing speech and images. AI Agents Work Together In 2025, we will see a significant shift from relying on individual AI models to using systems where multiple AI agents of diverse expertise work together. As an example, we recently introduced the\xa0Virtual Lab, where a professor AI agent leads a team of AI scientist agents (e.g., AI chemist, AI biologist) to tackle challenging, open-ended research, with a human researcher providing high-level feedback. We will experience an emerging paradigm of research around how humans work together with AI agents.'}]
===================
=== Final Answer ===
Here are some recent news articles discussing the future of AI agents in 2025:
1. **AI Agent Trends**
- **Source**: [Analytics Vidhya](https://www.analyticsvidhya.com/blog/2024/12/ai-agent-trends/)
- **Summary**: A study by Deloitte forecasts that 25% of enterprises using Generative AI will deploy AI agents by 2025, with this number expected to grow to 50% by 2027. Gartner predicts that by 2028, at least 15% of daily work decisions will be made autonomously through agentic AI.
2. **Next Year Will Be the Year of AI Agents**
- **Source**: [TechTarget](https://www.techtarget.com/searchEnterpriseAI/feature/Next-year-will-be-the-year-of-AI-agents)
- **Summary**: Analysts suggest that 2025 may focus on building the infrastructure necessary for AI agents. While single-task AI agents will rise, the development of a comprehensive orchestration layer across platforms will be crucial for their effectiveness.
3. **Predictions for AI in 2025**
- **Source**: [Stanford Institute for Human-Centered AI](https://hai.stanford.edu/news/predictions-ai-2025-collaborative-agents-ai-skepticism-and-new-risks)
- **Summary**: Experts predict a shift towards collaborative AI systems where multiple specialized agents work together, guided by humans. This includes the development of multimodal AI models in education and research, where AI agents collaborate on complex tasks.
These articles highlight the anticipated growth and evolution of AI agents, emphasizing collaboration, infrastructure development, and the integration of AI into enterprise decision-making processes.
====================
Modify the callback function to use it.
from typing import Dict, Any, Callable
# 1. Define AgentCallbacks class
class AgentCallbacks:
def __init__(
self,
tool_callback: Callable,
observation_callback: Callable,
result_callback: Callable,
):
self.tool_callback = tool_callback
self.observation_callback = observation_callback
self.result_callback = result_callback
# 2. Define AgentStreamParser class
class AgentStreamParser:
def __init__(self, callbacks: AgentCallbacks):
self.callbacks = callbacks
def process_agent_steps(self, step: Dict[str, Any]) -> None:
if "actions" in step:
for action in step["actions"]:
self.callbacks.tool_callback(
{"tool": action.tool, "tool_input": action.tool_input}
)
elif "output" in step:
self.callbacks.result_callback(step["output"])
else:
self.callbacks.observation_callback(step)
# 3. Define callback functions
def tool_callback(tool) -> None:
print("<<<<<<< Tool Called >>>>>>")
print(f"Tool: {tool.get('tool')}")
print(f"Input: {tool.get('tool_input')}")
print("<<<<<<< Tool Called >>>>>>")
def observation_callback(step) -> None:
print("<<<<<<< Observation >>>>>>")
observation_data = step["steps"][0].observation
print(f"Observation: {observation_data}")
print("<<<<<<< Observation >>>>>>")
def result_callback(result: str) -> None:
print("<<<<<<< Final Answer >>>>>>")
print(result)
print("<<<<<<< Final Answer >>>>>>")
# 4. Example usage
# Wrap callback functions into AgentCallbacks instance
agent_callbacks = AgentCallbacks(
tool_callback=tool_callback,
observation_callback=observation_callback,
result_callback=result_callback,
)
# Create AgentStreamParser instance
agent_stream_parser = AgentStreamParser(agent_callbacks)
Check the output content. You can reflect the output value of your callback functions, providing intermediate content that has been changed.
# Request streaming output for the query
result = agent_executor.stream({"input": "Search news about AI Agent in 2025."})
for step in result:
# Output intermediate steps using parser
agent_stream_parser.process_agent_steps(step)
<<<<<<< Tool Called >>>>>>
Tool: search_news
Input: {'query': 'AI Agent 2025'}
<<<<<<< Tool Called >>>>>>
<<<<<<< Observation >>>>>>
Observation: [{'url': 'https://www.analyticsvidhya.com/blog/2024/12/ai-agents-to-look-out-for/', 'content': "Q2. Why are these five AI agents considered game-changers for 2025? Ans. The selected agents—Oracle's Miracle Agent, Nvidia's Eureka Agent, Google's Project Jarvis, SAP's Joule Collaborative AI Agents, and Cisco's Webex AI Agent—stand out due to their innovative designs, broad applications, and industry impact."}, {'url': 'https://www.analyticsvidhya.com/blog/2024/12/ai-agent-trends/', 'content': 'In a similar study, Deloitte forecasts that 25% of enterprises using GenAI will deploy AI Agents by 2025, growing to 50% by 2027. Meanwhile, Gartner predicts that by 2028, at least 15% of day-to-day work decisions will be made autonomously through agentic AI. It also states that by then, 33% of enterprise software applications will also include'}, {'url': 'https://hai.stanford.edu/news/predictions-ai-2025-collaborative-agents-ai-skepticism-and-new-risks', 'content': 'According to leading experts from Stanford Institute for Human-Centered AI, one major trend is the rise of collaborative AI systems where multiple specialized agents work together, with humans providing high-level guidance. I expect to see more focus on multimodal AI models in education, including in processing speech and images. AI Agents Work Together In 2025, we will see a significant shift from relying on individual AI models to using systems where multiple AI agents of diverse expertise work together. As an example, we recently introduced the\xa0Virtual Lab, where a professor AI agent leads a team of AI scientist agents (e.g., AI chemist, AI biologist) to tackle challenging, open-ended research, with a human researcher providing high-level feedback. We will experience an emerging paradigm of research around how humans work together with AI agents.'}]
<<<<<<< Observation >>>>>>
<<<<<<< Final Answer >>>>>>
Here are some recent news articles discussing AI Agents and their expected developments in 2025:
1. **AI Agents to Look Out For**
- **Source**: [Analytics Vidhya](https://www.analyticsvidhya.com/blog/2024/12/ai-agents-to-look-out-for/)
- **Summary**: This article highlights five AI agents considered game-changers for 2025, including Oracle's Miracle Agent, Nvidia's Eureka Agent, Google's Project Jarvis, SAP's Joule Collaborative AI Agents, and Cisco's Webex AI Agent. These agents are noted for their innovative designs and broad applications across various industries.
2. **AI Agent Trends**
- **Source**: [Analytics Vidhya](https://www.analyticsvidhya.com/blog/2024/12/ai-agent-trends/)
- **Summary**: A study by Deloitte forecasts that 25% of enterprises using Generative AI will deploy AI Agents by 2025, with this number expected to grow to 50% by 2027. Gartner predicts that by 2028, at least 15% of daily work decisions will be made autonomously through AI agents.
3. **Predictions for AI in 2025**
- **Source**: [Stanford Institute for Human-Centered AI](https://hai.stanford.edu/news/predictions-ai-2025-collaborative-agents-ai-skepticism-and-new-risks)
- **Summary**: Experts predict a rise in collaborative AI systems where multiple specialized agents work together, guided by humans. The article discusses the shift from individual AI models to systems where diverse AI agents collaborate, exemplified by a Virtual Lab where an AI professor leads a team of AI scientist agents.
These articles provide insights into the anticipated advancements and trends in AI agents by 2025.
<<<<<<< Final Answer >>>>>>
Communicating Agent with previous conversation history
To remember past conversations, you can wrap the AgentExecutor
with RunnableWithMessageHistory
.
For more details on RunnableWithMessageHistory
, please refer to the link below.
Reference
from langchain_community.chat_message_histories import ChatMessageHistory
from langchain_core.runnables.history import RunnableWithMessageHistory
# Create a dictionary to store session_id
store = {}
# Function to get session history based on session_id
def get_session_history(session_ids):
if session_ids not in store: # If session_id is not in store
# Create a new ChatMessageHistory object and store it in store
store[session_ids] = ChatMessageHistory()
return store[session_ids] # Return session history for the corresponding session_id
# Create an agent with chat message history
agent_with_chat_history = RunnableWithMessageHistory(
agent_executor,
# Chat session_id
get_session_history,
# The key for the question input in the prompt: "input"
input_messages_key="input",
# The key for the message input in the prompt: "chat_history"
history_messages_key="chat_history",
)
# Request streaming output for the query
response = agent_with_chat_history.stream(
{"input": "Hello! My name is Teddy!"},
# Set session_id
config={"configurable": {"session_id": "abc123"}},
)
# Check the output
for step in response:
agent_stream_parser.process_agent_steps(step)
<<<<<<< Final Answer >>>>>>
Hello Teddy! How can I assist you today?
<<<<<<< Final Answer >>>>>>
# Request streaming output for the query
response = agent_with_chat_history.stream(
{"input": "What is my name?"},
# Set session_id
config={"configurable": {"session_id": "abc123"}},
)
# Check the output
for step in response:
agent_stream_parser.process_agent_steps(step)
<<<<<<< Final Answer >>>>>>
Your name is Teddy!
<<<<<<< Final Answer >>>>>>
# Request streaming output for the query
response = agent_with_chat_history.stream(
{
"input": "My email address is teddy@teddynote.com. The company name is TeddyNote Co., Ltd."
},
# Set session_id
config={"configurable": {"session_id": "abc123"}},
)
# Check the output
for step in response:
agent_stream_parser.process_agent_steps(step)
<<<<<<< Final Answer >>>>>>
Thank you for sharing that information, Teddy! How can I assist you with TeddyNote Co., Ltd. or anything else today?
<<<<<<< Final Answer >>>>>>
# Request streaming output for the query
response = agent_with_chat_history.stream(
{
"input": "Search the latest news and write it as the body of the email. "
"The recipient is `Ms. Sally` and the sender is my personal information."
"Write in a polite tone, and include appropriate greetings and closings at the beginning and end of the email."
},
# Set session_id
config={"configurable": {"session_id": "abc123"}},
)
# Check the output
for step in response:
agent_stream_parser.process_agent_steps(step)
<<<<<<< Tool Called >>>>>>
Tool: search_news
Input: {'query': 'latest news'}
<<<<<<< Tool Called >>>>>>
<<<<<<< Observation >>>>>>
Observation: [{'url': 'https://apnews.com/', 'content': '[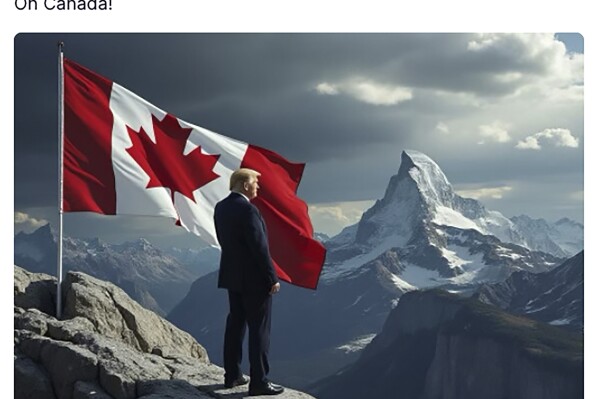](https://apnews.com/article/trump-trolling-canada-jill-biden-trudeau-39ecae6554c7b4350e6a106354672eb4) [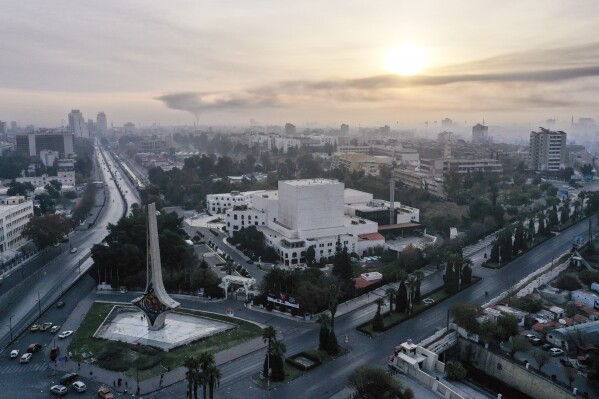](https://apnews.com/article/syria-israel-airstrike-assad-war-b90edb8dbe8268dacf90e59ca601e2e3) [](https://apnews.com/article/migrant-health-care-dreamers-lawsuit-504c8f05b3d93538cb7292318c267441) [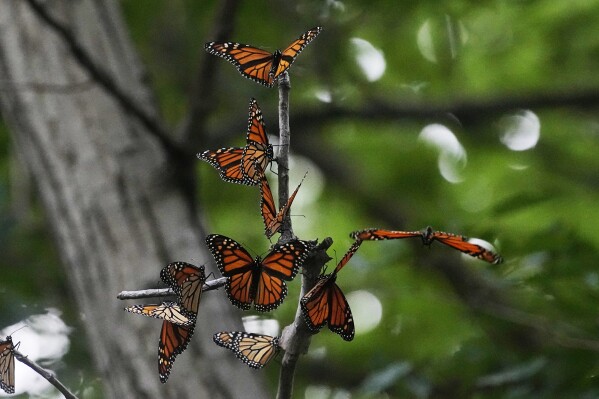](https://apnews.com/article/monarch-butterflies-endangered-species-climate-habitat-f5d4844289ede7b3d76918cc6f98a5cc) [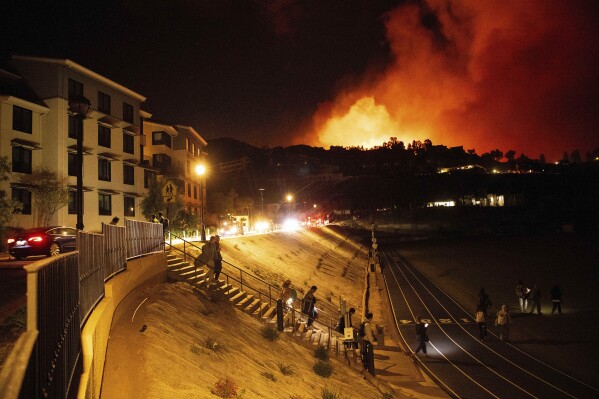](https://apnews.com/article/wildfire-malibu-evacuation-pepperdine-university-ef9f6ea11815be64feaf2e5e5eb7588c) [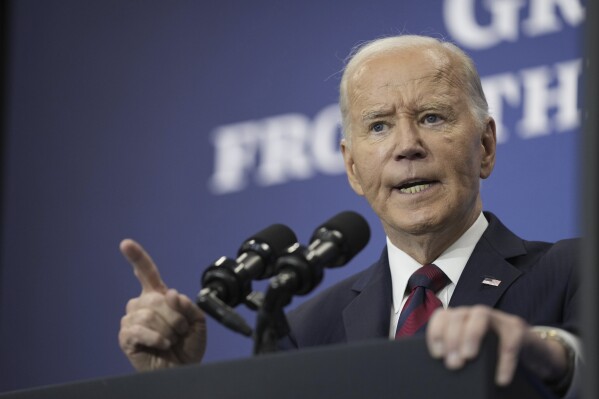](https://apnews.com/article/biden-trump-economy-stupid-jobs-inflation-cde78b58c6b4ccbcb7ce237f741e06a6) [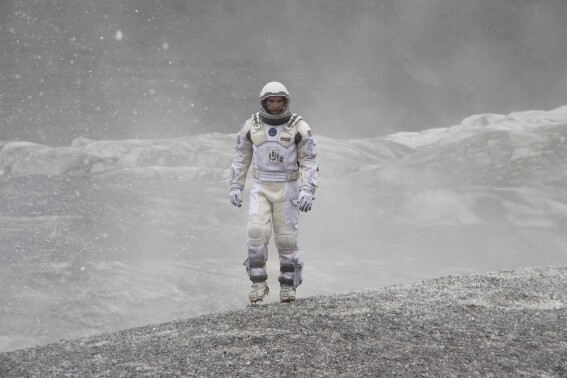](https://apnews.com/article/christopher-nolan-interstellar-rerelease-interview-bd7f4de84525062fb0d0e89a7fe6ea92) [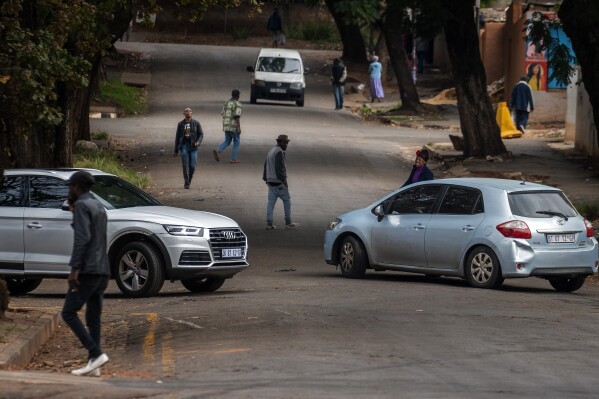](https://apnews.com/article/south-africa-car-road-crash-accident-2f063819764ae42cd4c8ffd5c76e4c6d) [](https://apnews.com/article/scrim-new-orleans-fugitive-dog-6fdddcb2cb694981f50f4c25ecfab655) [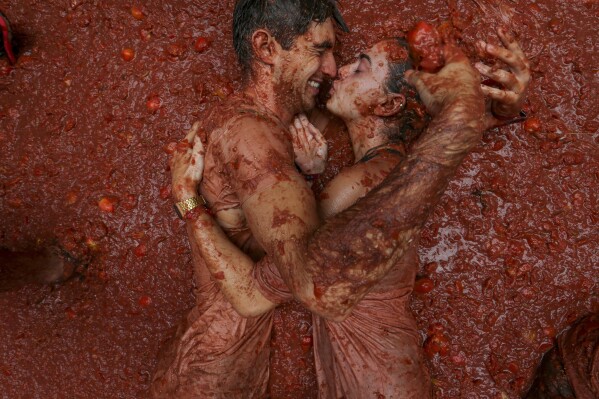](https://apnews.com/associated-press-100-photos-of-2024-an-epic-catalog-of-humanity) [](https://apnews.com/world-news/general-news-1637ffe66255f7048734759ec6bd99e9) [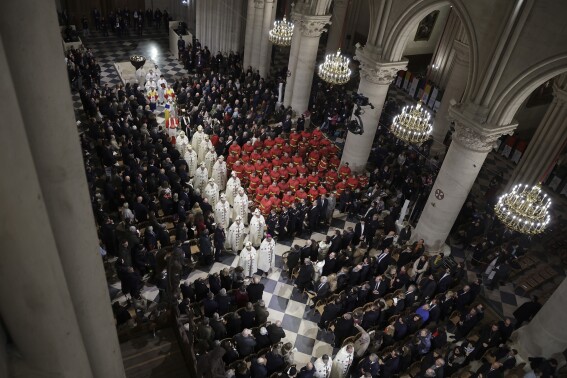](https://apnews.com/article/france-paris-notre-dame-carpenter-fire-catholic-ea516112b8393a795cd97b6abe5ef4e3) [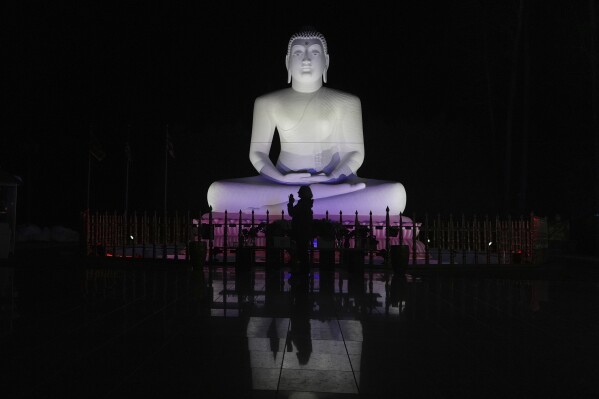](https://apnews.com/article/buddhism-interfaith-new-jersey-spirituality-ecce3b0d5569e0497c47c92eb57f9abc) [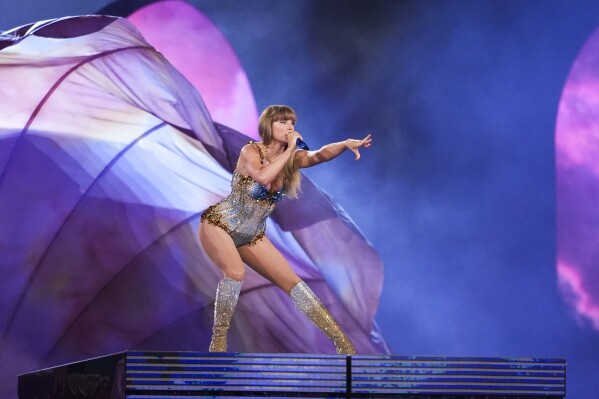](https://apnews.com/world-news/taylor-swift-general-news-domestic-news-add8d87cc892eac2838c51812ea032de) [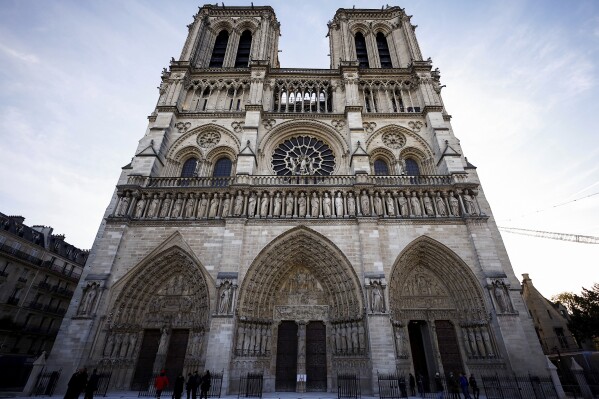](https://apnews.com/article/notre-dame-cathedral-reopening-fire-paris-28cf9686d7d48dce0c046ca9e46f3e99) [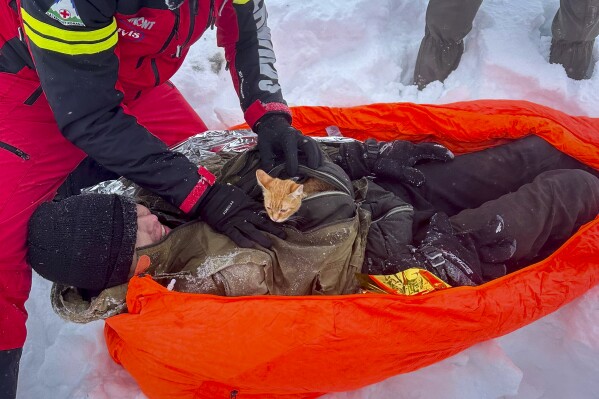](https://apnews.com/article/romania-ukraine-draft-cat-rescue-mountain-war-8e57bc8b07a2ec80ea16ca9cd09450b3) [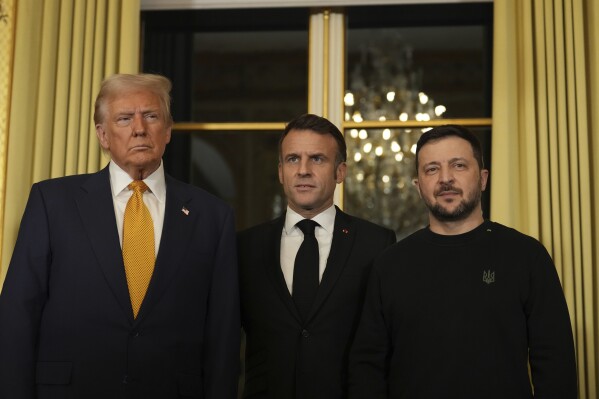](https://apnews.com/article/trump-paris-notre-dame-f97fde62ca2ce68c3874c395b305e26b) [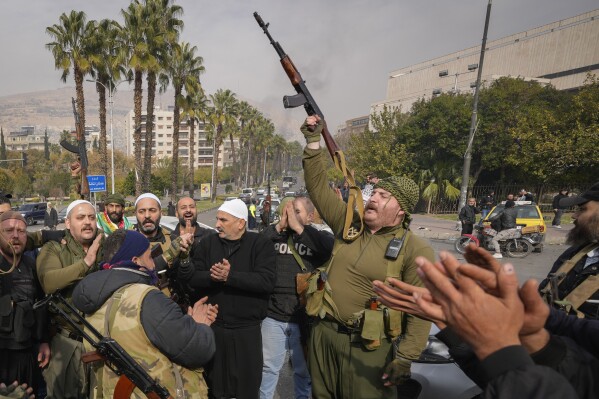](https://apnews.com/article/syria-assad-ousted-rebel-offensive-timeline-8c54a8b97803d4b10cde53b97227128e) [](https://apnews.com/article/trump-ukraine-military-aid-russia-biden-efbcff8ed068e621055e8fa70b5905e1) [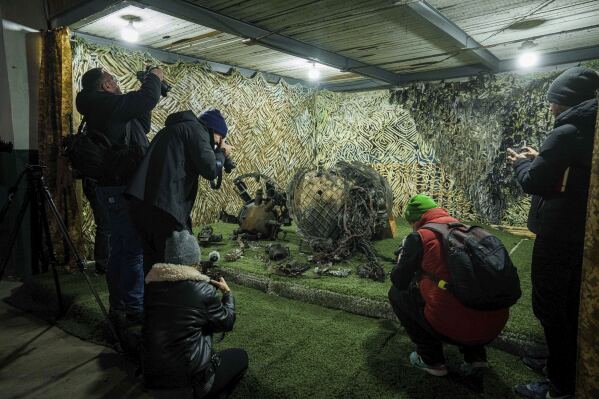](https://apnews.com/article/russia-oreshnik-hypersonic-missile-putin-ukraine-war-345588a399158b9eb0b56990b8149bd9) [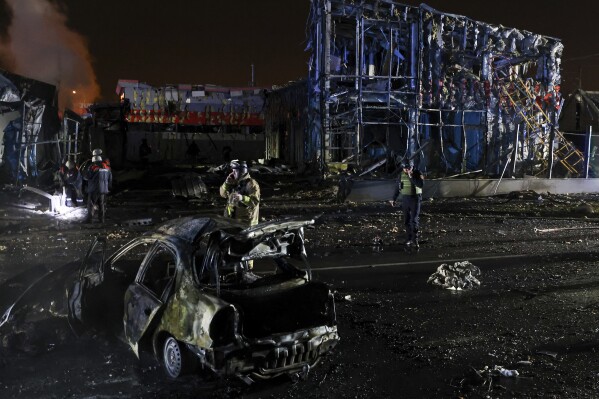](https://apnews.com/article/russia-ukraine-war-zelenskyy-troops-nato-9bf883670879e2a398fe1a7ebfb71ddb) [](https://apnews.com/article/russia-putin-syria-assad-ukraine-war-31fa9b933372b3704ed285c96863892b) [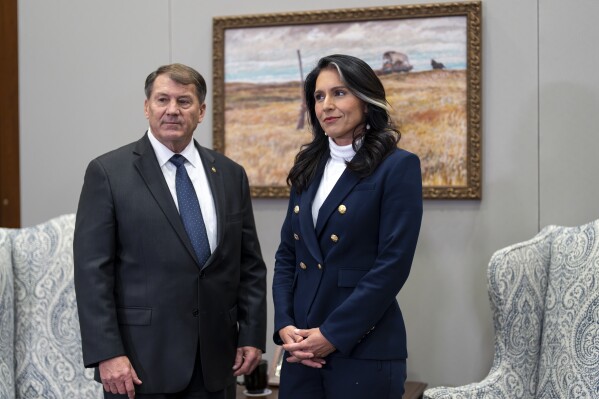](https://apnews.com/article/tulsi-gabbard-hegseth-kash-patel-trump-syria-44d0150e7d251946b60fc7f6799c4a74) [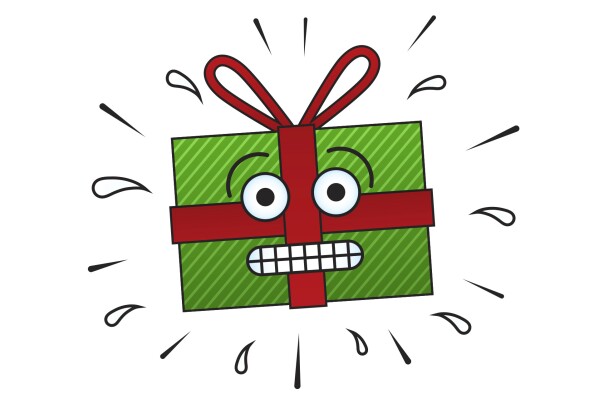](https://apnews.com/article/secret-santa-stress-holiday-gift-guide-d5a907bd8aa1b0b1ebf1e04bba9fe89c) [](https://apnews.com/article/november-project-exercise-social-fitness-winter-fc55e4aefbd003f999aafec15fe25a0e) [](https://apnews.com/article/personal-trainer-exercise-workout-c94f4f6625d2d6a5a77537946d1518b5) [](https://apnews.com/article/travel-stress-holiday-tips-db5e5d819b6251bd415a9ad204baa03a) [](https://apnews.com/article/work-life-stress-reduction-breathing-techniques-8c0636a09d605ef0c56e529e8be0f2f9) [](https://apnews.com/article/ap-top-asia-photos-of-2024-e071bc96deed1052511a53e0e1cc61f9) [](https://apnews.com/article/argentina-milei-trump-musk-default-economy-inflation-libertarian-18efe55d81df459792a038ea9e321800) [](https://apnews.com/article/ap-sports-photos-of-year-d07f4a6c90716b4e5e46df43cea695e8) [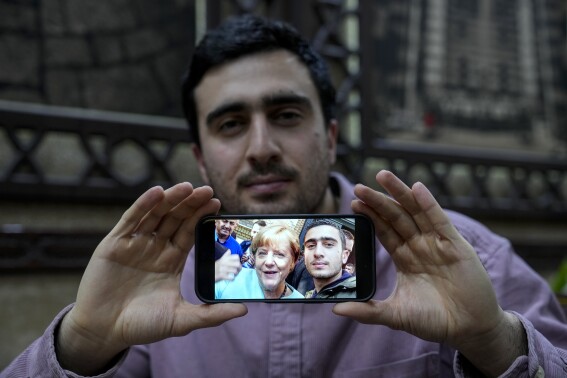](https://apnews.com/article/germany-syria-refugees-asylum-future-ab24be8f2ffef4e118ed12a4b0df110f) [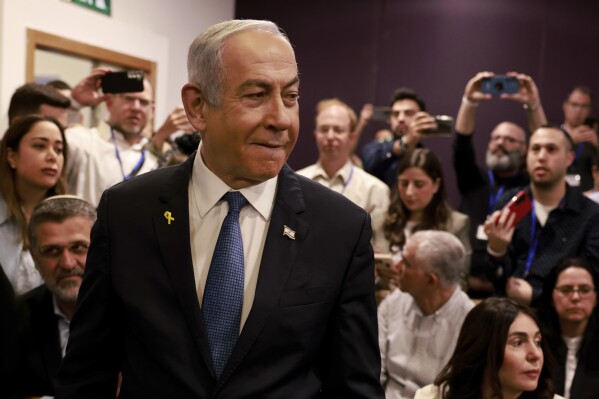](https://apnews.com/article/israel-netanyahu-corruption-trial-gaza-478c957c7749986d5b8b2d039f670d54) [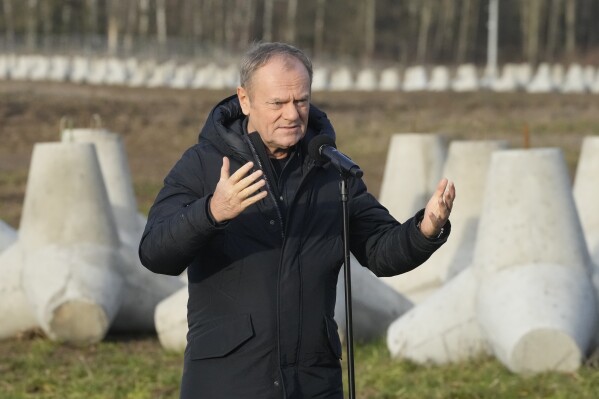](https://apnews.com/article/ukraine-war-poland-tusk-negotiations-russia-eu-23802e5b26f5c0c1d45a32e9cc80f362) [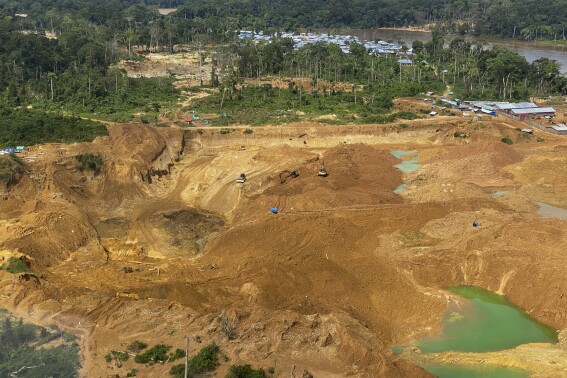](https://apnews.com/article/congo-world-heritage-site-gold-mining-china-5e9499fd939c3c2d798a6165f3fc487b) [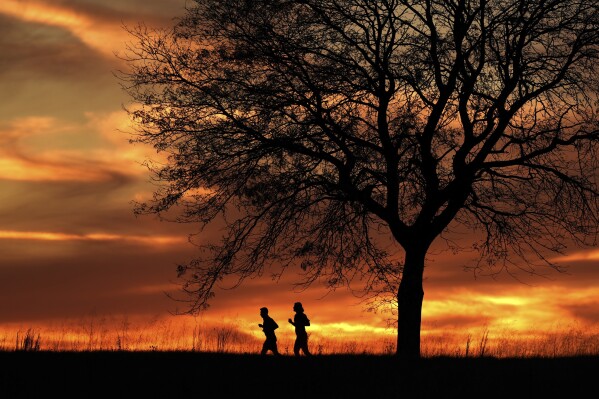](https://apnews.com/article/climate-change-november-hottest-year-record-d1f41a2c7341c006f051ad321915d89c) [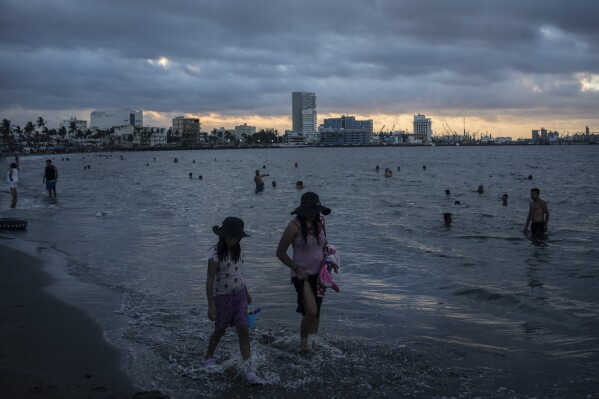](https://apnews.com/article/deadly-heat-humidity-mexico-climate-change-2e8db903deabd015f608e45204a19bf0) [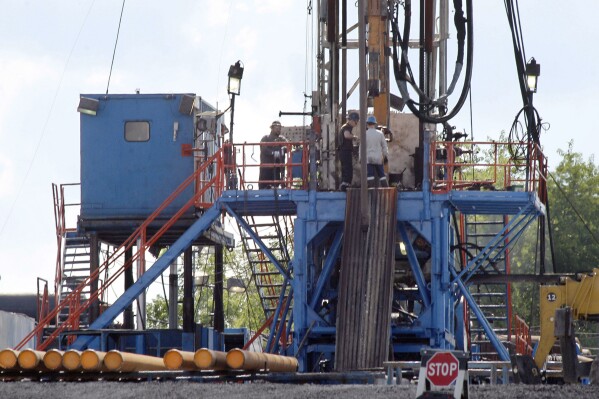](https://apnews.com/article/trump-energy-permitting-reform-drilling-billion-investment-dd99706a325082cdf475e599ec3c0687) [](https://apnews.com/article/supreme-court-environment-regulation-recuse-gorsuch-utah-16cff708f7549ff430823213eeaa22f6) [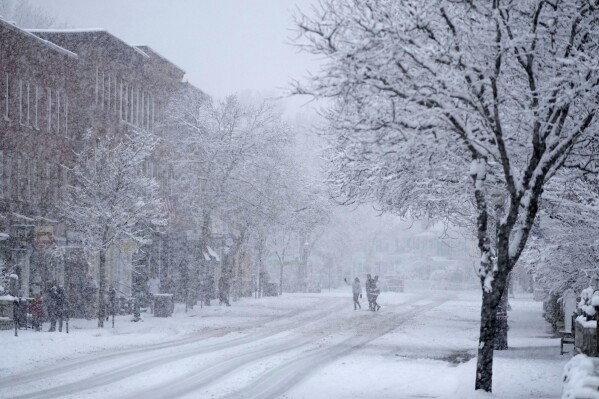](https://apnews.com/article/fact-check-vermont-supreme-court-vaccination-ruling-0256fc7ad888230ee8dc51f6fe0d479a)'}, {'url': 'https://www.cnn.com/', 'content': 'View the latest news and breaking news today for U.S., world, weather, entertainment, politics and health at CNN.com.'}, {'url': 'https://www.foxnews.com/', 'content': "[](https://www.foxbusiness.com/politics/trump-makes-vow-anyone-who-invests-1-billion-more-us) [](https://www.foxnews.com/media/democratic-governors-refuse-say-how-old-too-old-2028-nominee) [](https://www.foxnews.com/politics/meet-natalie-harp-trumps-valuable-resource-who-lawmakers-say-critical-his-operation) [](https://www.foxnews.com/politics/trump-shooting-task-force-says-dhs-secret-service-havent-produced-docs-golf-course-incident) [Video](https://www.foxnews.com/video/6365813443112) [](https://www.foxnews.com/media/border-crisis-top-issue-many-want-trump-address-his-first-100-days-office) [](https://www.foxnews.com/politics/biden-says-trump-inheriting-strongest-economy-modern-history-slams-tariff-plan-major-mistake)"}]
<<<<<<< Observation >>>>>>
<<<<<<< Final Answer >>>>>>
Here's a draft for your email to Ms. Sally, including the latest news:
---
**Subject:** Latest News Update
Dear Ms. Sally,
I hope this message finds you well.
I wanted to share some of the latest news highlights that may interest you:
1. **Trump's Investment Vow**: Former President Trump has made a significant promise to anyone who invests $1 billion or more in the United States. [Read more here.](https://www.foxbusiness.com/politics/trump-makes-vow-anyone-who-invests-1-billion-more-us)
2. **Democratic Governors on Age Limits**: Democratic governors have chosen not to address the age limit for future presidential nominees, raising questions about the party's direction. [Read more here.](https://www.foxnews.com/media/democratic-governors-refuse-say-how-old-too-old-2028-nominee)
3. **Meet Trump's 'Valuable Resource'**: A profile on Natalie Harp, who is considered a critical resource for Trump's operations, has been released. [Read more here.](https://www.foxnews.com/politics/meet-natalie-harp-trumps-valuable-resource-who-lawmakers-say-critical-his-operation)
4. **Biden's Comments on the Economy**: President Biden has stated that Trump is inheriting the "strongest economy in modern history," while criticizing his tariff plan as a major mistake. [Read more here.](https://www.foxnews.com/politics/biden-says-trump-inheriting-strongest-economy-modern-history-slams-tariff-plan-major-mistake)
For more updates, you can visit [CNN](https://www.cnn.com/) or [AP News](https://apnews.com/).
Thank you for your attention, and I look forward to hearing your thoughts.
Best regards,
Teddy
teddy@teddynote.com
TeddyNote Co., Ltd.
---
Feel free to modify any part of the email as you see fit!
<<<<<<< Final Answer >>>>>>
# Request streaming output for the query
response = agent_with_chat_history.stream(
{"input": "What is my name?"},
# Set session_id
config={"configurable": {"session_id": "def456"}},
)
# Check the output
for step in response:
agent_stream_parser.process_agent_steps(step)
<<<<<<< Final Answer >>>>>>
I don't have access to your personal information, so I don't know your name. If you'd like to share it, feel free!
<<<<<<< Final Answer >>>>>>
Last updated